Android Downlod Google Drive File using Java
Google Drive provides a powerful API that allows you to programmatically interact with your files and folders in the cloud. In this tutorial, we will demonstrate how to download
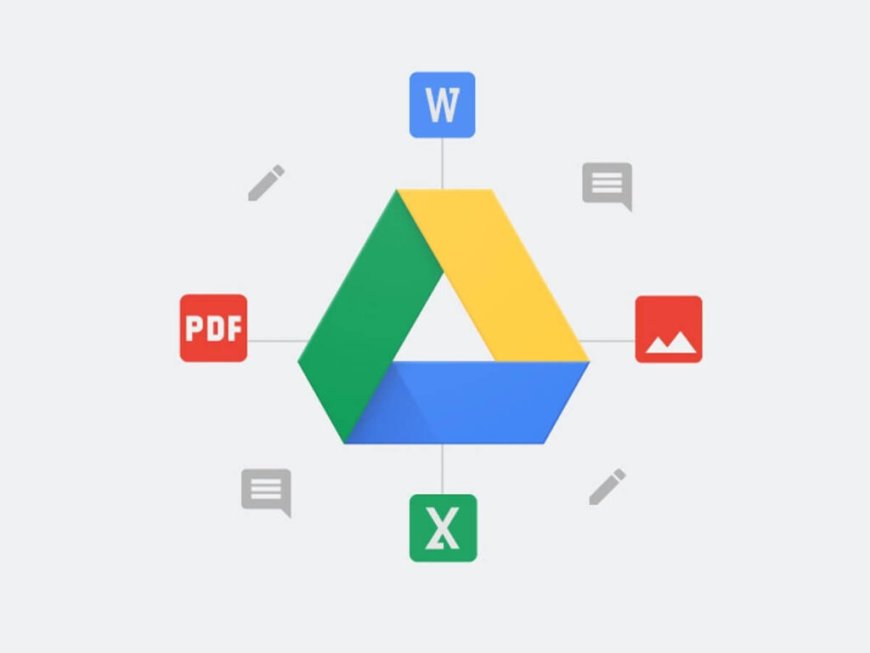
Google Drive provides a powerful API that allows you to programmatically interact with your files and folders in the cloud. In this tutorial, we will demonstrate how to download a file from Google Drive by specifying its ID using Java and the Google Drive API client library.
Prerequisites
Before we begin, make sure you have the following prerequisites in place:
-
Google Developer Account: You need a Google Developer account to create a project and enable the Google Drive API. You can create one at Google Developer Console.
-
Java Development Environment: Ensure that you have Java installed on your system and set up a development environment. You can download Java from the official website.
-
Google Drive API Project: Create a new project in the Google Developer Console and enable the Google Drive API for that project. You will also need to create credentials and download a JSON file containing the client ID and secret. Make sure to enable billing for your project to access Google Drive API.
Setting Up Your Java Project
To get started, you'll need to set up a Java project and include the Google Drive API client library as a dependency. You can use Maven or Gradle for this purpose. Here, we'll use Maven:
-
Create a new Maven project or open an existing one.
-
Open your project's
pom.xml
file and add the following dependencies:<dependencies> <dependency> <groupId>com.google.api-client</groupId> <artifactId>google-api-client</artifactId> <version>1.32.1</version> <!-- Check for the latest version on the Google API Client library page --> </dependency> <dependency> <groupId>com.google.oauth-client</groupId> <artifactId>google-oauth-client</artifactId> <version>1.35.0</version> <!-- Check for the latest version on the Google OAuth Client library page --> </dependency> <dependency> <groupId>com.google.apis</groupId> <artifactId>google-api-services-drive</artifactId> <version>v3-rev305-1.25.0</version> <!-- Check for the latest version on the Google Drive API library page --> </dependency> </dependencies>
- Save the
pom.xml
file, and your project should automatically download the required dependencies.
Authenticating Your Application
To access Google Drive, you need to authenticate your Java application using OAuth 2.0. Follow these steps:
-
Place the JSON file containing your OAuth 2.0 client ID and secret (downloaded from the Google Developer Console) in a secure location.
-
Load the credentials file in your Java code using a
GoogleCredential
object. Replace"YOUR_CLIENT_ID.json"
with the actual name of your credentials file:import com.google.api.client.googleapis.auth.oauth2.GoogleCredential; import com.google.api.services.drive.Drive; import com.google.api.services.drive.DriveScopes; public class DriveDownloader { private static Drive driveService; public static void main(String[] args) { try { // Load the credentials JSON file GoogleCredential credentials = GoogleCredential.fromStream(new FileInputStream("YOUR_CLIENT_ID.json")) .createScoped(Collections.singleton(DriveScopes.DRIVE)); // Create a Drive service instance driveService = new Drive.Builder( GoogleNetHttpTransport.newTrustedTransport(), JacksonFactory.getDefaultInstance(), credentials) .setApplicationName("Google Drive Downloader") .build(); // Your download code will go here } catch (Exception e) { e.printStackTrace(); } } }
Downloading a File by ID
Now that you have set up the authentication, you can proceed to download a file from Google Drive by specifying its ID. Here's how to do it:
import com.google.api.client.http.GenericUrl;
import com.google.api.client.http.HttpResponse;
import com.google.api.client.http.HttpResponseException;
public class DriveDownloader {
// ... (authentication setup code)
public static void main(String[] args) {
try {
// ... (authentication code)
// Specify the file ID of the file you want to download
String fileId = "YOUR_FILE_ID_HERE";
// Specify the local file path where you want to save the downloaded file
String localFilePath = "path/to/save/downloaded/file.txt";
// Download the file
downloadFileById(fileId, localFilePath);
System.out.println("File downloaded successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
private static void downloadFileById(String fileId, String localFilePath) throws IOException {
// Create a URL for downloading the file
GenericUrl downloadUrl = new GenericUrl("https://www.googleapis.com/drive/v3/files/" + fileId);
// Create an HTTP request to download the file
HttpResponse response = driveService.getRequestFactory().buildGetRequest(downloadUrl).execute();
// Check if the response is successful
if (response.isSuccessStatusCode()) {
// Get the content stream from the response
InputStream inputStream = response.getContent();
// Create a FileOutputStream to save the downloaded file locally
FileOutputStream outputStream = new FileOutputStream(localFilePath);
// Read and write the file content
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
// Close the streams
inputStream.close();
outputStream.close();
} else {
throw new HttpResponseException(response.getStatusCode(), response.getStatusMessage());
}
}
}
In this code:
- Replace
"YOUR_FILE_ID_HERE"
with the actual ID of the file you want to download. - Specify the local file path where you want to save the downloaded file.
- The
downloadFileById
method sends an HTTP GET request to the Google Drive API to retrieve the file's content and then saves it locally.
Running the Application
With your Java project set up, OAuth 2.0 authentication configured, and the download code in place, you are ready to run your application. When you execute your Java program, it will download the specified file from Google Drive to your local machine.
Conclusion
In this tutorial, you learned how to download a file from Google Drive by specifying its ID using Java and the Google Drive API client library. By following the steps outlined above, you can create Java applications that interact with Google Drive, allowing you to automate file downloads, uploads, and various other tasks in your Google Drive account.