Implementing A Two-way Sync For Google Drive In Java
Implementing a two-way sync for Google Drive in Java involves using the Google Drive API, handling file comparisons, and ensuring that changes are propagated in both directions. Below
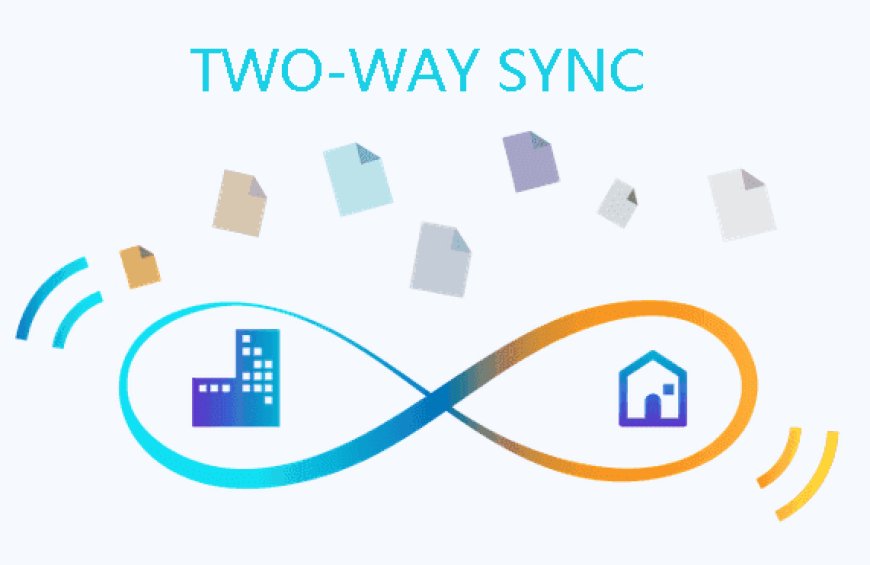
Google Drive is a popular cloud storage service that allows users to store and share files online. It provides a convenient way to access files from anywhere and collaborate with others. However, there may be situations where you need to synchronize files between your local machine and Google Drive.
Implementing a two-way sync for Google Drive in Java involves developing a Java application that can synchronize files and folders between a local directory and a Google Drive account. This synchronization process should be bidirectional, meaning changes made in either the local directory or the Google Drive account should be reflected in both locations. This can be achieved by utilizing the Google Drive API, which provides methods for accessing and manipulating files and folders in Google Drive. By integrating this API into a Java application, developers can create a robust and efficient two-way sync solution for Google Drive.
The Google Drive API provides a set of methods and classes that allow developers to interact with Google Drive programmatically. By leveraging this API, developers can build applications that can synchronize files between a local machine and Google Drive seamlessly.
To implement a two-way sync, we need to follow the below steps:
Step 1: Setting up the Google Drive API
To get started, you will need to set up the Google Drive API in your Java project. First, you need to create a new project in the Google Cloud Console and enable the Google Drive API. Once you have done that, you will need to generate a client ID and client secret, which will be used to authenticate your application with Google Drive.
Step 2: Authenticating with Google Drive
To authenticate your application with Google Drive, you will need to use the OAuth 2.0 protocol. This involves obtaining an access token that allows your application to access the user's Google Drive. You can use the Google OAuth Client Library for Java to simplify the authentication process.
Step 3: Retrieving the list of files from Google Drive
Once you have authenticated your application, you can start retrieving the list of files from Google Drive. The Google Drive API provides a convenient way to search for files using various criteria such as file name, file type, and modification date. You can use the Files.list() method to retrieve a list of files that match your search criteria.
Step 4: Comparing the local files with the files on Google Drive
To implement a two-way sync, you need to compare the files on your local machine with the files on Google Drive. You can use the java.io.File class to retrieve the list of files from a local directory. Once you have the list of local files and the list of files from Google Drive, you can compare them based on their file names, sizes, and modification dates.
Step 5: Uploading new or modified files to Google Drive
If you find any new or modified files on your local machine, you will need to upload them to Google Drive. You can use the Files.create() method to create a new file on Google Drive. To upload the file, you will need to read the file content and send it to Google Drive using the Files.update() method.
Step 6: Downloading new or modified files from Google Drive
If you find any new or modified files on Google Drive, you will need to download them to your local machine. You can use the Files.get() method to retrieve the file content from Google Drive. Once you have the file content, you can write it to a local file using the java.io.FileOutputStream class.
Step 7: Deleting files from Google Drive
If you find any files on Google Drive that are not present on your local machine, you will need to delete them from Google Drive. You can use the Files.delete() method to delete a file from Google Drive.
Step 8: Handling conflicts
In some cases, you may encounter conflicts where a file has been modified both on your local machine and on Google Drive. To handle conflicts that may arise when a file is modified on both sides, we can use a conflict resolution strategy. This strategy can be as simple as choosing the most recently modified version of the file or as complex as merging the changes from both versions. The choice of strategy depends on the specific requirements of the application.
Please note that this example provides a basic framework and should be extended to handle various edge cases and error handling.
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.DriveScopes;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
import com.google.api.client.googleapis.auth.oauth2.GoogleCredential;
import java.io.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class TwoWayDriveSync {
private static Drive driveService;
public static void main(String[] args) throws IOException {
// Set up Google Drive API client
driveService = initializeDriveService();
// Define local and remote folders
String localFolderPath = "/path/to/local/folder";
String remoteFolderId = "your_remote_folder_id";
// Perform two-way sync
syncLocalToRemote(localFolderPath, remoteFolderId);
syncRemoteToLocal(localFolderPath, remoteFolderId);
}
private static Drive initializeDriveService() throws IOException {
GoogleCredential credential = new GoogleCredential.Builder()
.setTransport(httpTransport)
.setJsonFactory(jsonFactory)
.setServiceAccountId("your-service-account-email@developer.gserviceaccount.com")
.setServiceAccountPrivateKeyFromP12File(new File("path/to/your/privatekey.p12"))
.setServiceAccountScopes(Collections.singleton(DriveScopes.DRIVE))
.build();
return new Drive.Builder(httpTransport, jsonFactory, credential).setApplicationName("Your Application Name").build();
}
private static void syncLocalToRemote(String localFolderPath, String remoteFolderId) throws IOException {
// List local files
List<File> localFiles = listLocalFiles(localFolderPath);
// List remote files
List<File> remoteFiles = listRemoteFiles(remoteFolderId);
// Compare and upload missing local files to remote
for (File localFile : localFiles) {
boolean fileExistsRemotely = false;
for (File remoteFile : remoteFiles) {
if (localFile.getName().equals(remoteFile.getName())) {
fileExistsRemotely = true;
break;
}
}
if (!fileExistsRemotely) {
// Upload localFile to remoteFolderId
uploadFile(localFile, remoteFolderId);
}
}
}
private static void syncRemoteToLocal(String localFolderPath, String remoteFolderId) throws IOException {
// List local files
List<File> localFiles = listLocalFiles(localFolderPath);
// List remote files
List<File> remoteFiles = listRemoteFiles(remoteFolderId);
// Compare and download missing remote files to local
for (File remoteFile : remoteFiles) {
boolean fileExistsLocally = false;
for (File localFile : localFiles) {
if (remoteFile.getName().equals(localFile.getName())) {
fileExistsLocally = true;
break;
}
}
if (!fileExistsLocally) {
// Download remoteFile to localFolderPath
downloadFile(remoteFile, localFolderPath);
}
}
}
private static List<File> listLocalFiles(String localFolderPath) {
// Implement logic to list local files in the specified folder
// Return a list of local File objects
// You can use java.io.File or any other library to handle local files
return new ArrayList<>();
}
private static List<File> listRemoteFiles(String remoteFolderId) throws IOException {
FileList result = driveService.files().list()
.setQ("'" + remoteFolderId + "' in parents")
.setFields("files(id, name)")
.execute();
return result.getFiles();
}
private static void uploadFile(File localFile, String remoteFolderId) throws IOException {
// Implement logic to upload a local file to the specified remote folder
}
private static void downloadFile(File remoteFile, String localFolderPath) throws IOException {
// Implement logic to download a remote file to the specified local folder
}
}
In this example:
- Initialize the Google Drive API client.
- List local and remote files.
- Compare the files and perform the necessary actions to sync files in both directions.
Please note that you will need to set up a service account and download the necessary JSON key file and add the required dependencies for the Google Drive API client library to your project. Additionally, error handling and edge cases are simplified in this example and should be extended for a production-ready application.