Command Line Arguments in C
Command line arguments allow you to pass information to a C program when it is executed in the terminal
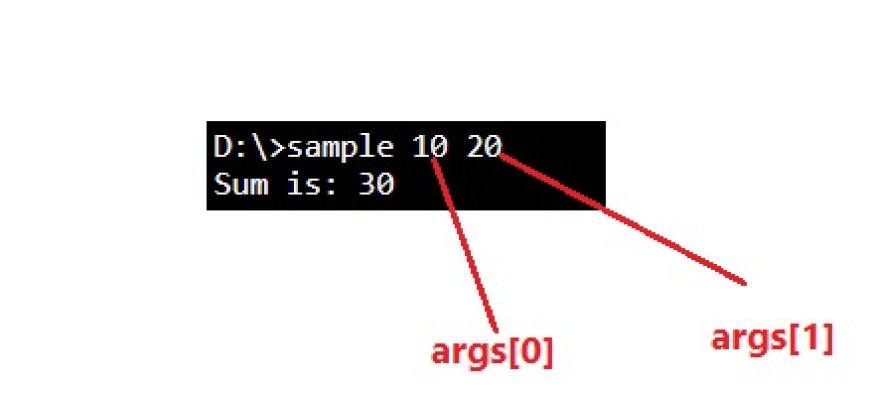
Command line arguments allow you to pass information to a C program when it is executed in the terminal. They provide a way to make your programs more flexible and interactive by enabling users to customize their behavior without modifying the source code. In this tutorial, we'll explore command line arguments in C, including their usage, handling, and examples.
1. Introduction to Command Line Arguments
Command line arguments are values that are provided to a program when it is executed in the terminal or command prompt. These arguments are typically used to configure the program's behavior or provide input data. They are separated by spaces and follow the program's name in the command line.
For example:
./myprogram arg1 arg2 arg3
In this example, arg1
, arg2
, and arg3
are command line arguments passed to the program myprogram
.
2. How to Access Command Line Arguments
In C, command line arguments are accessed through the main
function's arguments. The main
function has two parameters:
int main(int argc, char *argv[])
argc
(argument count): An integer representing the number of command line arguments, including the program name itself.argv
(argument vector): An array of strings (char*
) containing the actual command line arguments.
Here's a simple example of how to access and print command line arguments:
#include <stdio.h>
int main(int argc, char *argv[]) {
// Check if at least one argument (program name) is provided
if (argc < 2) {
printf("Usage: %s <arg1> <arg2> ...\n", argv[0]);
return 1; // Exit with an error code
}
printf("Program name: %s\n", argv[0]);
// Loop through and print all arguments (starting from argv[1])
for (int i = 1; i < argc; i++) {
printf("Argument %d: %s\n", i, argv[i]);
}
return 0;
}
3. Example Programs
Let's explore two example programs to demonstrate the practical usage of command line arguments.
3.1. Simple Argument Parsing
This program takes two integer arguments and performs addition.
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
// Check if exactly three arguments are provided
if (argc != 3) {
printf("Usage: %s <num1> <num2>\n", argv[0]);
return 1; // Exit with an error code
}
// Convert arguments to integers
int num1 = atoi(argv[1]);
int num2 = atoi(argv[2]);
// Perform addition and display the result
int sum = num1 + num2;
printf("Sum: %d\n", sum);
return 0;
}
Usage:
./addition 5 7
3.2. Calculator Program
This program implements a basic calculator that can perform addition, subtraction, multiplication, and division operations.
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
// Check if at least four arguments are provided
if (argc != 4) {
printf("Usage: %s <num1> <operator> <num2>\n", argv[0]);
return 1; // Exit with an error code
}
// Convert arguments to numbers
double num1 = atof(argv[1]);
char operator = argv[2][0]; // Get the first character of the operator string
double num2 = atof(argv[3]);
// Perform the requested operation
double result;
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
// Check for division by zero
if (num2 == 0) {
printf("Error: Division by zero is not allowed.\n");
return 1;
}
result = num1 / num2;
break;
default:
printf("Error: Invalid operator '%c'\n", operator);
return 1;
}
printf("Result: %.2lf\n", result);
return 0;
}
Usage:
./calculator 5 + 3
./calculator 10 / 2
./calculator 7 ^ 2
4. Tips and Best Practices
- Always check the number of command line arguments (
argc
) to ensure that the correct number of arguments are provided. - Convert command line arguments to the appropriate data types using functions like
atoi
(for integers) andatof
(for floating-point numbers). - Handle invalid or unexpected inputs gracefully and provide informative error messages to the user.
- Document the expected command line arguments and usage of your program.
5. Conclusion
Command line arguments in C provide a convenient way to make your programs interactive and configurable. By understanding how to access and use command line arguments, you can create more versatile and user-friendly command line applications. Remember to handle errors and edge cases to ensure the robustness of your programs.