Variable Arguments in C
Header files in C have the extension .h and typically contain declarations of functions, data types, constants, and macros that are used in one or more source files (.c files).
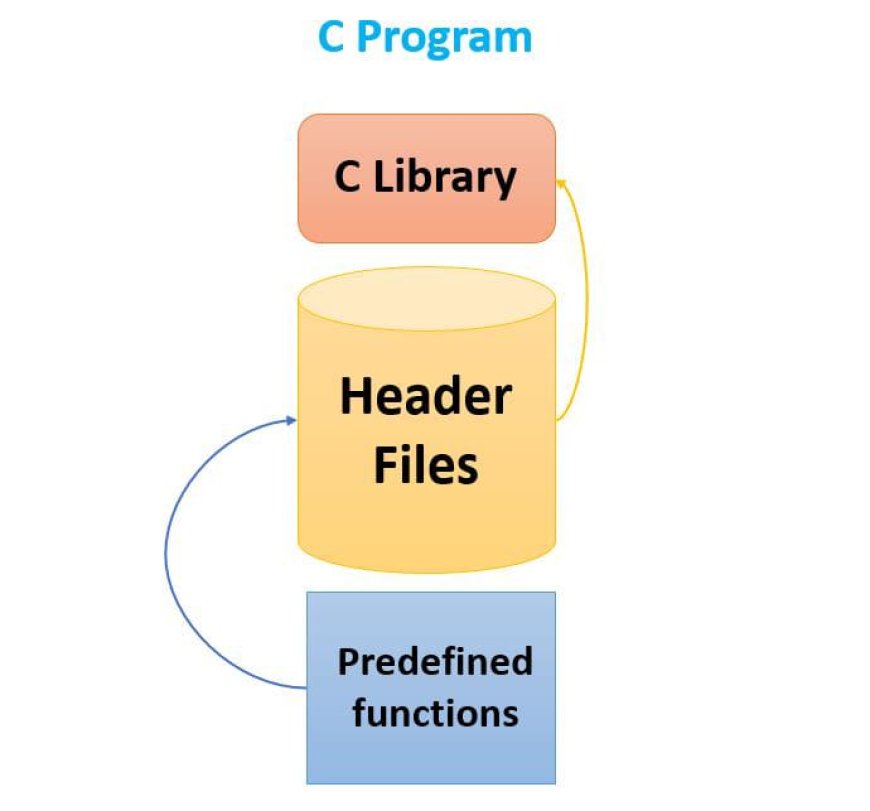
Header files in C play a crucial role in code organization, modularity, and reusability. They allow you to declare functions, data structures, and constants that can be used across multiple source files. In this tutorial, we will explore what header files are, how to create and use them, and provide examples to illustrate their usage.
1. Introduction to Header Files
Header files in C have the extension .h
and typically contain declarations of functions, data types, constants, and macros that are used in one or more source files (.c
files). Header files help in separating the interface (declarations) of a module or library from its implementation (definitions). This separation promotes code reuse and makes large programs more manageable.
2. Creating a Header File
A header file usually consists of the following elements:
- Include Guards: To prevent multiple inclusions.
- Function Prototypes: Declarations of functions defined in a corresponding source file.
- Data Type Definitions: Declarations of structures, enums, and other custom data types.
- Global Constants and Macros: Declarations of constants and macros.
Here's an example of a simple header file named myheader.h
:
#ifndef MYHEADER_H
#define MYHEADER_H
// Function prototypes
int add(int a, int b);
int subtract(int a, int b);
// Constants
#define PI 3.14159265359
#endif
3. Including Header Files
To use a header file in your C program, you need to include it using the #include
preprocessor directive. Typically, this is done at the beginning of your source file. For example:
#include "myheader.h"
When you include a header file, its declarations become visible in the source file, allowing you to use the functions, data types, constants, and macros defined in the header.
4. Header Guards
Header guards (or include guards) are used to prevent a header file from being included multiple times in the same source file or in multiple source files. They ensure that the contents of the header file are included only once, avoiding duplicate symbol declarations and potential compilation errors.
The #ifndef
, #define
, and #endif
preprocessor directives are used to create header guards, as shown in the example header file above.
5. Example: Creating and Using a Header File
Let's create a simple program that uses the myheader.h
header file:
main.c:
#include <stdio.h>
#include "myheader.h" // Include our custom header file
int main() {
int result = add(5, 3);
printf("5 + 3 = %d\n", result);
result = subtract(10, 4);
printf("10 - 4 = %d\n", result);
printf("The value of PI: %f\n", PI);
return 0;
}
Compile and Run:
gcc main.c -o myprogram
./myprogram
In this example, we include our custom myheader.h
header file, which provides function prototypes for add
and subtract
as well as the constant PI
. The main program can use these declarations without needing to know their implementations.
6. Common C Standard Library Header Files
Besides creating custom header files, C provides a set of standard library header files that offer a wide range of functionality. Some common standard library headers include:
<stdio.h>
: Input and output functions.<stdlib.h>
: Memory allocation and general utilities.<string.h>
: String manipulation functions.<math.h>
: Mathematical functions and constants.<time.h>
: Date and time functions.
You can include these headers to access the functions and constants they define. For example, including <stdio.h>
allows you to use functions like printf
and scanf
.
In summary, header files in C are essential for organizing code, promoting modularity, and facilitating code reuse. They provide a clear separation between interface and implementation, making it easier to collaborate on large projects and maintain codebases.