How to Request Runtime Permissions in Android
Runtime permissions have become a critical aspect of Android development. They provide a way for users to have more control over their privacy and data.
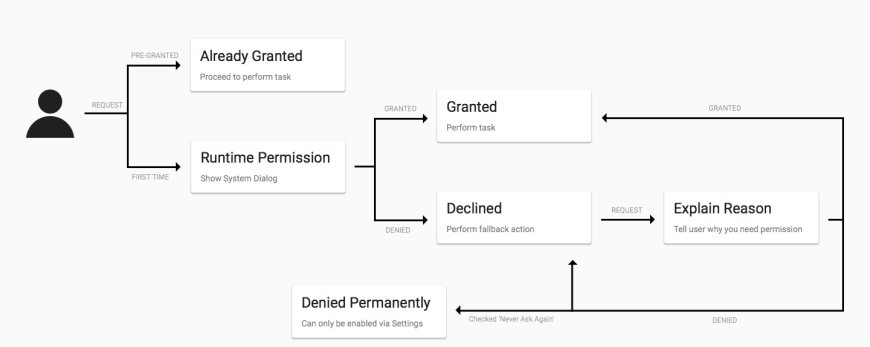
Android is a versatile and powerful operating system that powers a vast array of mobile devices. To make the most out of these devices, Android applications often need access to various device resources such as the camera, location, and contacts. However, Android prioritizes user privacy and security, which is why developers are required to request runtime permissions from the user before accessing these resources. In this article, we will explore how to request runtime permissions in Android using Java with practical examples.
Understanding Runtime Permissions
Before Android 6.0 (API level 23), Android applications could request permissions during installation. Users had to grant all requested permissions at once, which often led to privacy concerns. Starting from Android 6.0, the Android operating system introduced the concept of runtime permissions, allowing users to grant or deny specific permissions when the application attempts to use them.
Runtime permissions have become a critical aspect of Android development. They provide a way for users to have more control over their privacy and data. As a developer, it's crucial to request these permissions gracefully and handle user responses effectively.
Step 1: Define Permissions in the Manifest
Before you can request runtime permissions, you must declare them in your AndroidManifest.xml file. The following is an example of how to declare the CAMERA permission:
<uses-permission android:name="android.permission.CAMERA" />
You should include all the permissions your app requires in this file.
Step 2: Checking Permission Status
Before requesting a permission, it's a good practice to check if the permission has already been granted. You can do this using the PackageManager
class. Here's an example of how to check for the CAMERA permission:
// Check if the CAMERA permission is granted
int cameraPermission = ContextCompat.checkSelfPermission(this, Manifest.permission.CAMERA);
if (cameraPermission == PackageManager.PERMISSION_GRANTED) {
// Permission is granted, proceed with using the camera
} else {
// Permission is not granted, request it from the user
}
Step 3: Requesting Permissions
When the permission is not granted, you need to request it from the user. To request permissions, you should use the requestPermissions
method. Here's how you can request the CAMERA permission:
// Request CAMERA permission
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.CAMERA}, CAMERA_PERMISSION_CODE);
In the above code snippet, CAMERA_PERMISSION_CODE
is an arbitrary integer constant that you can define to identify the permission request when the user responds.
Step 4: Handling Permission Results
After you request permissions, the user will be presented with a permission dialog. They can choose to grant or deny the permission. To handle the user's response, you need to override the onRequestPermissionsResult
method in your activity or fragment:
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if (requestCode == CAMERA_PERMISSION_CODE) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// CAMERA permission is granted, you can now use the camera
} else {
// CAMERA permission is denied, handle the situation gracefully
}
}
}
In the above code, we check if the requestCode matches the one we used when requesting the permission. Then, we check if the permission was granted or denied based on the grantResults
array.
Putting It All Together - A Camera Permission Example
Let's put everything we've learned into a practical example of requesting the CAMERA permission and using the camera when it's granted.
import android.Manifest;
import android.content.pm.PackageManager;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import androidx.core.content.ContextCompat;
public class MainActivity extends AppCompatActivity {
private static final int CAMERA_PERMISSION_CODE = 101;
private Button openCameraButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
openCameraButton = findViewById(R.id.openCameraButton);
openCameraButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int cameraPermission = ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.CAMERA);
if (cameraPermission == PackageManager.PERMISSION_GRANTED) {
// CAMERA permission is granted, proceed with using the camera
openCamera();
} else {
// CAMERA permission is not granted, request it from the user
ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.CAMERA}, CAMERA_PERMISSION_CODE);
}
}
});
}
private void openCamera() {
// TODO: Implement camera functionality here
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if (requestCode == CAMERA_PERMISSION_CODE) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// CAMERA permission is granted, you can now use the camera
openCamera();
} else {
// CAMERA permission is denied, handle the situation gracefully
}
}
}
}