Preprocessors in C
The C preprocessor is a part of the C compiler that handles directives beginning with a # symbol and manipulate source code before it's compiled.
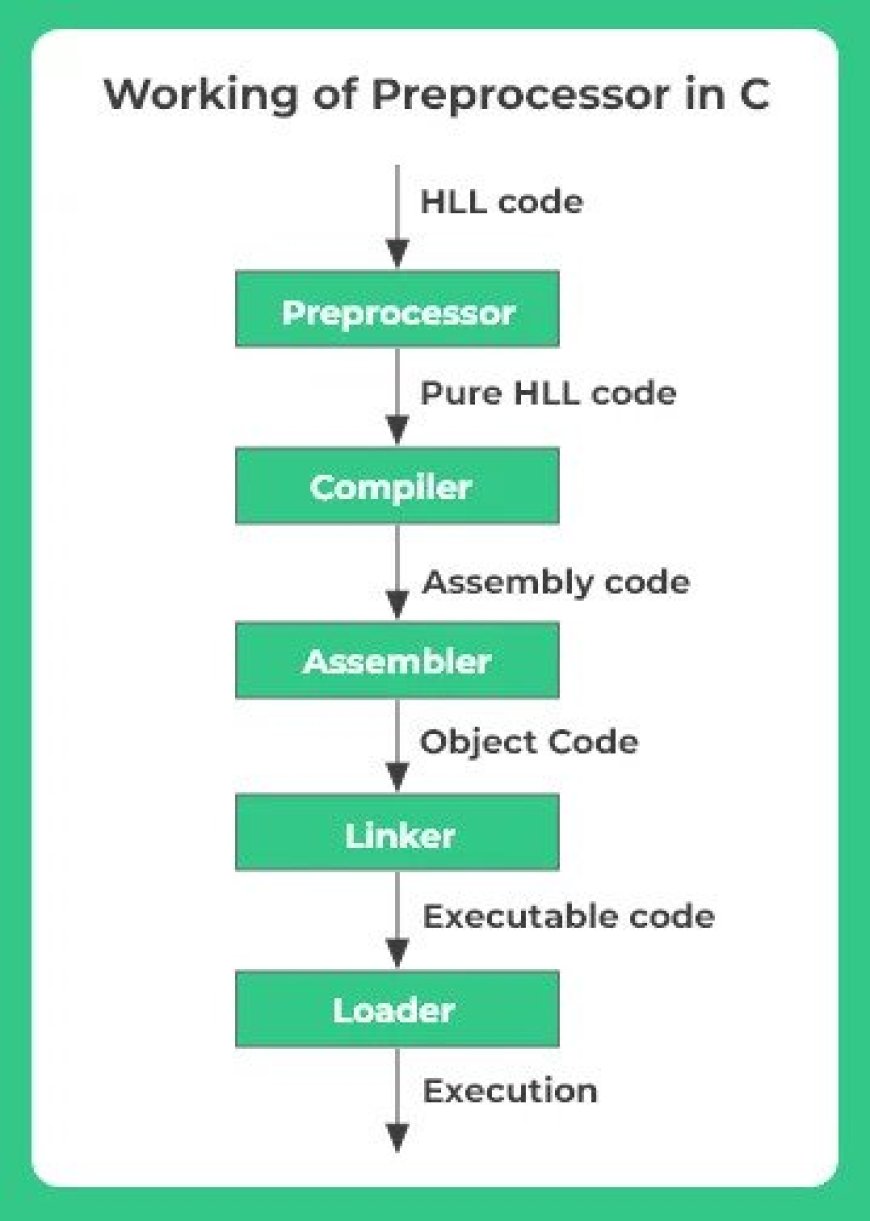
Preprocessors in C are tools that manipulate your source code before it's compiled. They enable you to perform various tasks such as including header files, defining constants, and performing conditional compilation. In this tutorial, we'll explore preprocessors in C, including their usage, directives, and examples.
1. Introduction to Preprocessors
The C preprocessor is a part of the C compiler that handles directives beginning with a #
symbol. These directives are processed before the actual compilation begins. Preprocessors are used to modify or generate C code and can significantly enhance code readability, reusability, and maintainability.
2. Common Preprocessor Directives
2.1. #include
The #include
directive is used to include the contents of header files in your code. This allows you to reuse code from other files.
#include <stdio.h> // Include the standard I/O header
#include "myheader.h" // Include a user-defined header
2.2. #define
The #define
directive is used to create macros and constants. Macros are like functions that get replaced with code during preprocessing.
#define MAX_VALUE 100 // Define a constant
#define SQUARE(x) ((x) * (x)) // Define a macro
2.3. #ifdef
, #ifndef
, #else
, and #endif
These directives are used for conditional compilation. You can include or exclude code based on predefined macros.
#ifdef DEBUG
// Debug-specific code
#else
// Release code
#endif
2.4. #pragma
The #pragma
directive is used to give special instructions to the compiler. It's often used for compiler-specific optimizations and settings.
#pragma pack(1) // Specify byte alignment
3. Example Programs
Let's explore some example programs to demonstrate the use of preprocessors in C.
3.1. Conditional Compilation
In this example, we use conditional compilation to include different code sections based on whether the DEBUG
macro is defined or not.
#include <stdio.h>
#define DEBUG
int main() {
#ifdef DEBUG
printf("Debug mode enabled.\n");
#else
printf("Release mode enabled.\n");
#endif
return 0;
}
Compile without the -DDEBUG
flag for release mode, and with it for debug mode.
3.2. Macros and Constants
Here, we define a constant and a macro to calculate the square of a number.
#include <stdio.h>
#define MAX_VALUE 100
#define SQUARE(x) ((x) * (x))
int main() {
int num = 5;
if (num > MAX_VALUE) {
printf("Number exceeds the maximum value.\n");
}
int square = SQUARE(num);
printf("The square of %d is %d.\n", num, square);
return 0;
}
3.3. Including Header Files
In this example, we use the #include
directive to include a user-defined header file.
// myheader.h
#ifndef MYHEADER_H
#define MYHEADER_H
void myFunction();
#endif
// main.c
#include <stdio.h>
#include "myheader.h"
int main() {
printf("Hello, world!\n");
myFunction();
return 0;
}
4. Tips and Best Practices
- Use header guards (
#ifndef
,#define
, and#endif
) in header files to prevent multiple inclusion. - Keep your preprocessor directives organized and well-documented.
- Use meaningful names for macros and constants to enhance code readability.
- Avoid complex macros that can lead to unexpected behavior.
5. Conclusion
Preprocessors in C are a powerful tool for code organization, reuse, and conditional compilation. By using preprocessor directives like #include
, #define
, and conditional statements, you can create more modular and maintainable C code. However, it's essential to use these features judiciously and follow best practices to avoid code complexity and maintain code readability.