Memory Management in C
Memory management in C is the process of allocating and releasing memory resources for program data and variables.
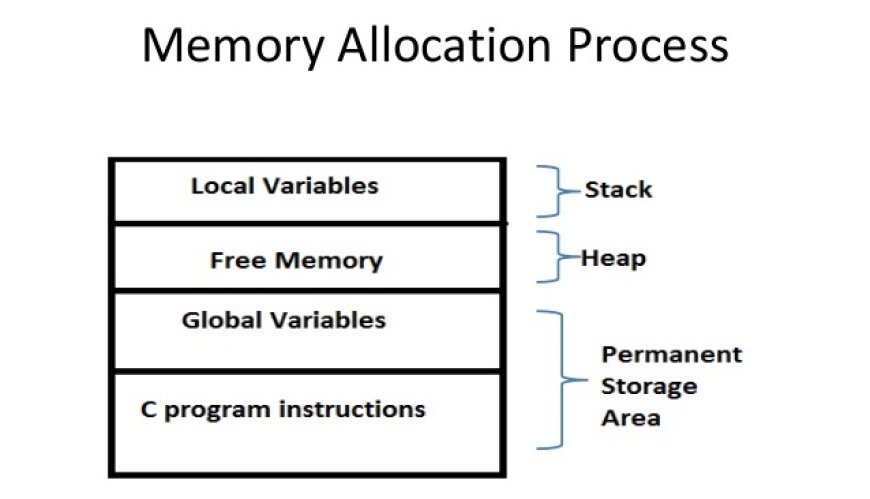
Memory management is a critical aspect of programming in the C language. It involves allocating and deallocating memory for various data structures like arrays, structs, and pointers. Understanding memory management is crucial to prevent memory leaks, segmentation faults, and optimize your code's performance. In this tutorial, we'll cover the basics of memory management in C with examples.
1. Introduction to Memory Management
Memory management in C is the process of allocating and releasing memory resources for program data and variables. Memory in a C program is organized into different regions:
- Stack: Used for function call management and local variables.
- Heap: Used for dynamic memory allocation and management.
- Static/Data: Used for global and static variables.
In this tutorial, we will focus on dynamic memory management, which involves allocating and releasing memory from the heap.
2. Static vs. Dynamic Memory Allocation
-
Static Memory Allocation: Memory is allocated at compile-time. The size of the memory block is fixed and determined during code compilation. This is suitable for fixed-size data structures.
-
Dynamic Memory Allocation: Memory is allocated at runtime. The size of the memory block can vary depending on program requirements. This is useful for data structures with unknown or changing sizes.
3. Dynamic Memory Allocation Functions
C provides three primary functions for dynamic memory allocation:
malloc()
malloc(size_t size)
allocates a block of memory of the specifiedsize
in bytes.- Returns a pointer to the first byte of the allocated memory.
- If memory allocation fails, it returns
NULL
.
calloc()
calloc(size_t num_elements, size_t element_size)
allocates memory for an array ofnum_elements
each of sizeelement_size
bytes.- Initializes the allocated memory to zero.
- Returns a pointer to the first byte of the allocated memory.
- If memory allocation fails, it returns
NULL
.
realloc()
realloc(void* ptr, size_t new_size)
changes the size of the previously allocated memory block pointed to byptr
to the new sizenew_size
.- Returns a pointer to the resized memory block.
- If memory allocation fails, it returns
NULL
.
4. Memory Deallocation
To prevent memory leaks, it is essential to release dynamically allocated memory when it is no longer needed. C provides the free()
function for this purpose.
free()
free(void* ptr)
deallocates the memory block pointed to byptr
.- After calling
free()
, the memory can be reused for other allocations. - It is crucial to
free()
memory to avoid memory leaks.
5. Memory Leak Detection
Memory leaks occur when memory is allocated but never deallocated. Tools like Valgrind can help detect memory leaks in C programs. Always make sure to free()
dynamically allocated memory to prevent leaks.
6. Memory Management Examples
Let's go through two examples to illustrate memory management in C.
Example 1: Dynamic Array Allocation
#include <stdio.h>
#include <stdlib.h>
int main() {
int n;
printf("Enter the number of integers: ");
scanf("%d", &n);
// Allocate memory for an array of integers
int *arr = (int *)malloc(n * sizeof(int));
if (arr == NULL) {
printf("Memory allocation failed!");
return 1;
}
// Initialize the array
for (int i = 0; i < n; i++) {
arr[i] = i * 10;
}
// Print the array
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
// Deallocate memory
free(arr);
return 0;
}
In this example, we dynamically allocate memory for an integer array of size n
, initialize it, print its elements, and then free the allocated memory.
Example 2: Dynamic Struct Allocation
#include <stdio.h>
#include <stdlib.h>
struct Student {
char name[50];
int age;
};
int main() {
struct Student *s;
// Allocate memory for a struct
s = (struct Student *)malloc(sizeof(struct Student));
if (s == NULL) {
printf("Memory allocation failed!");
return 1;
}
// Initialize the struct
strcpy(s->name, "John");
s->age = 20;
// Print the struct members
printf("Name: %s\n", s->name);
printf("Age: %d\n", s->age);
// Deallocate memory
free(s);
return 0;
}
In this example, we allocate memory for a struct
and initialize its members before deallocating the memory.
7. Best Practices
- Always check if memory allocation with
malloc()
,calloc()
, orrealloc()
succeeds by verifying if the returned pointer is notNULL
. - Free dynamically allocated memory with
free()
when it is no longer needed to prevent memory leaks. - Avoid accessing memory after it has been freed; this leads to undefined behavior.
- Be mindful of buffer overflows and ensure that you don't write more data than allocated memory can hold.