Variables and Data Types in the C Language
A variable is a named storage location in a computer's memory that holds a value.This value can change during the course of a program's execution.
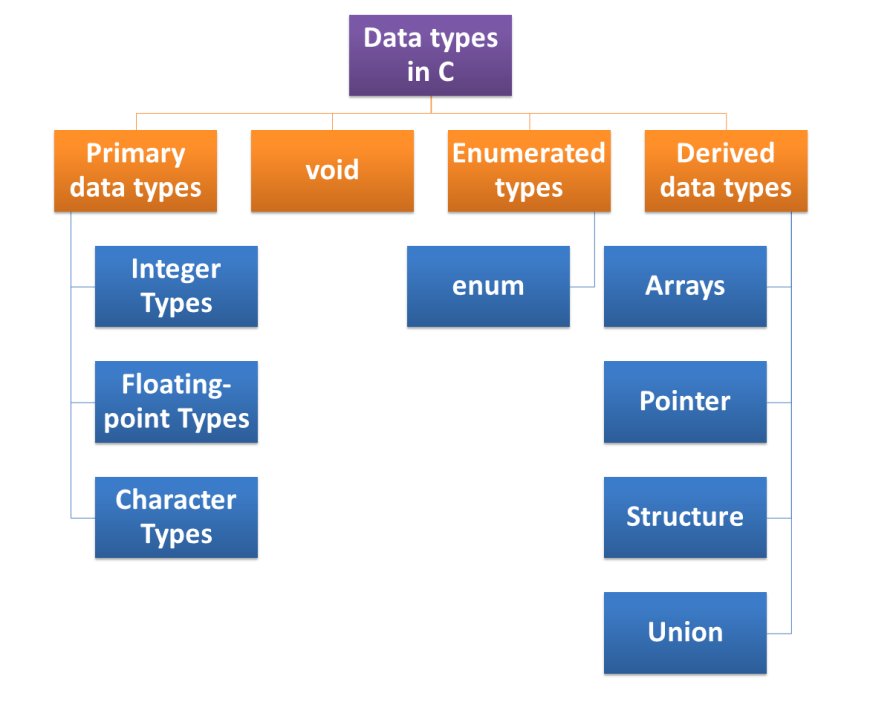
Programming languages serve as a means to communicate with computers and instruct them to perform specific tasks. In the world of programming, one fundamental concept is the utilization of variables and data types. These concepts are crucial for storing and manipulating different types of information within a program. In the C language, a powerful and widely-used programming language, understanding variables and data types is essential for writing efficient and reliable code.
Introduction to Variables
In programming, a variable is a named storage location in a computer's memory that holds a value. This value can change during the course of a program's execution. Variables provide a way to store and manage data, enabling programmers to work with dynamic and evolving information.
In C, variables must be declared before they are used. The declaration specifies the variable's name and its data type. The syntax for declaring a variable is as follows:
data_type variable_name;
Here, data_type
represents the type of data that the variable can hold, and variable_name
is the name assigned to the variable. For example:
int age;
float temperature;
char initial;
Common Data Types in C
C supports various data types, each tailored to specific kinds of data. Here are some of the common data types in C:
-
int: Represents integer values. It can store both positive and negative whole numbers.
-
float: Used to store single-precision floating-point numbers. This data type is suitable for values with decimal points.
-
double: Similar to
float
, but it stores double-precision floating-point numbers, which can hold more significant digits and provide higher precision. -
char: Holds a single character, such as a letter, digit, or symbol. Characters are enclosed in single quotes, e.g.,
'A'
,'3'
,'%'
. -
_Bool: Represents boolean values, which can be either
true
orfalse
. In C,0
is consideredfalse
, and any non-zero value is consideredtrue
. -
short and long: Modifiers that can be applied to
int
.short
reduces the range of values that theint
can hold, whilelong
extends it. For example,short int
andlong int
.
Modifiers and Qualifiers
C also provides various modifiers and qualifiers that can be applied to the basic data types:
-
signed and unsigned: These qualifiers modify integer types.
signed
allows both positive and negative values, whileunsigned
only allows positive values, effectively doubling the range of positive values. -
const: Declares a variable as constant, meaning its value cannot be changed after initialization.
-
volatile: Indicates that a variable can be changed by external factors that the program cannot predict, preventing certain optimizations by the compiler.
Variable Initialization
Variables can be assigned values at the time of declaration. This process is called initialization. For example:
int count = 10;
float pi = 3.14159;
char grade = 'A';
Conclusion
In the C programming language, variables and data types are fundamental concepts that serve as building blocks for creating powerful and efficient programs. Understanding the different data types, modifiers, and qualifiers is crucial for writing code that not only compiles correctly but also accurately represents the intended information.
By mastering variables and data types, programmers gain the ability to manage various kinds of information, from integers to characters to floating-point numbers. This knowledge forms the foundation upon which more complex programs and algorithms can be built. Whether you're a novice programmer or an experienced coder, a solid grasp of variables and data types is essential for harnessing the full potential of the C language.