Control Structures in C Language
They allow developers to make decisions, repeat actions, and control the logical sequence of operations. The C programming language provides a range of control structures
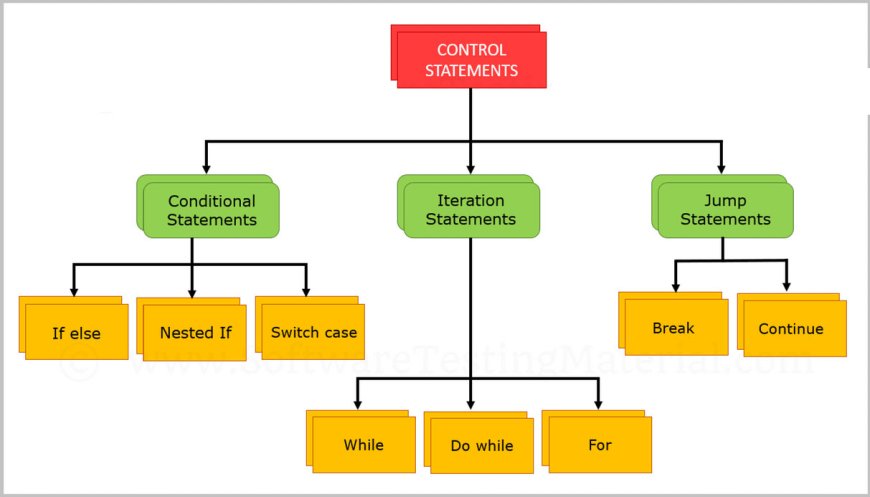
Control structures are essential components of programming that determine the flow of execution in a program. They allow developers to make decisions, repeat actions, and control the logical sequence of operations. The C programming language provides a range of control structures that enable programmers to create structured and efficient code. In this article, we explore the various control structures in C with illustrative examples.
1. Conditional Statements
Conditional statements are used to make decisions based on certain conditions. The most common conditional statement in C is the if
statement.
Example:
#include
int main() {
int num = 10;
if (num > 5) {
printf("The number is greater than 5.\n");
} else {
printf("The number is not greater than 5.\n");
}
return 0;
}
2. Loops
Loops are used to repeatedly execute a block of code as long as a certain condition is met. The two main types of loops in C are for
loops and while
loops.
Example (for loop):
#include
int main() {
for (int i = 1; i <= 5; i++) {
printf("Iteration %d\n", i);
}
return 0;
}
Example (while loop):
#include
int main() {
int count = 0;
while (count < 5) {
printf("Count: %d\n", count);
count++;
}
return 0;
}
Example (do-while loop):
#include
int main() {
int count = 0;
do {
printf("Count: %d\n", count);
count++;
}while (count < 5);
return 0;
}
4. Difference between for, while and do while loop
Sr. No | For loop | While loop | Do while loop |
---|---|---|---|
1. |
Syntax: For(initialization; condition;updating), { . Statements; } |
Syntax: While(condition), { . Statements; . } |
Syntax: Do { . Statements; } While(condition); |
2. | It is known as entry controlled loop | It is known as entry controlled loop. | It is known as exit controlled loop. |
3. | If the condition is not true first time than control will never enter in a loop | If the condition is not true first time than control will never enter in a loop. | Even if the condition is not true for the first time the control will enter in a loop. |
4. | There is no semicolon; after the condition in the syntax of the for loop. | There is no semicolon; after the condition in the syntax of the while loop. | There is semicolon; after the condition in the syntax of the do while loop. |
5. | Initialization and updating is the part of the syntax. | Initialization and updating is not the part of the syntax. | Initialization and updating is not the part of the syntax |
6. | For loop is used when we know the number of iterations means where the loop will terminate. | While loop is used when we don't know the number of iterations means where the loop will terminate. | Do while loop is used when we don't know the number of iterations means where the loop will terminate. |
3. Switch Statement
The switch
statement is used to select one of many code blocks to be executed, based on the value of an expression.
Example:
#include
int main() {
int day = 3;
switch (day) {
case 1:
printf("Sunday\n");
break;
case 2:
printf("Monday\n");
break;
case 3:
printf("Tuesday\n");
break;
default:
printf("Invalid day\n");
break;
}
return 0;
}
4. Control Statements
Control statements alter the normal flow of execution by allowing you to exit loops or skip iterations.
Example (break statement):
#include
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break;
}
printf("Iteration %d\n", i);
}
return 0;
}
Example (continue statement):
#include
int main() {
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue;
}
printf("Iteration %d\n", i);
}
return 0;
}
5. Nested Control Structures
Control structures can be nested within each other to create complex logic flows.
Example:
#include
int main() {
int rows = 5;
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= i; j++) {
printf("* ");
}
printf("\n");
}
return 0;
}
Conclusion
Control structures are the building blocks of structured programming in the C language. They provide the means to implement decision-making, looping, and altering program execution. By combining different control structures, developers can create sophisticated programs that perform tasks ranging from simple calculations to intricate algorithmic operations. Understanding and effectively utilizing control structures is essential for writing efficient, readable, and functional C code that meets various programming challenges.