typedef in C
typedef allows you to define new names for existing data types in C. It is especially useful when working with complex data structures like structs and unions,
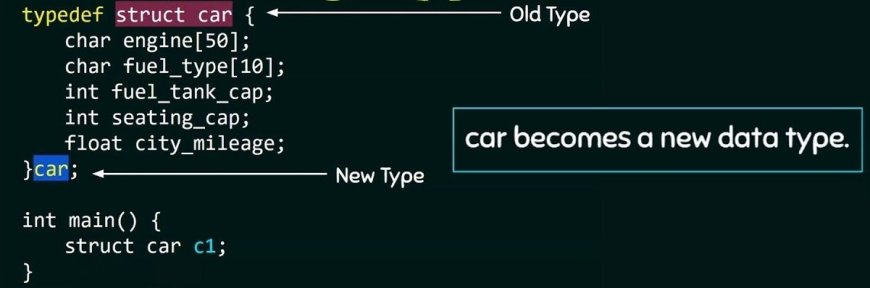
The typedef
keyword in C is used to create user-defined data types, which provide a way to create more meaningful, self-documenting type names for existing data types. This tutorial will explain the concept of typedef
, its syntax, and provide examples to illustrate its use.
1. Introduction to typedef
typedef
allows you to define new names for existing data types in C, making your code more readable and expressive. It is especially useful when working with complex data structures like structs and unions, or when you want to create descriptive type names for built-in types.
2. Syntax of typedef
The syntax of typedef
is straightforward:
typedef existing_type new_type_name;
existing_type
: The existing data type that you want to assign a new name to.new_type_name
: The new name you want to create for the existing data type.
Here's a simple example:
typedef int myInt; // Creates a new name "myInt" for the "int" data type
3. Using typedef
with Structures and Unions
typedef
becomes particularly useful when defining new type names for complex data structures like structs and unions. This allows you to create more concise and self-explanatory code. For example:
typedef struct {
int x;
int y;
} Point; // Creates a new name "Point" for an anonymous struct
In this case, we've created a new type name "Point" for an anonymous struct that contains two integers, x
and y
. Now, you can use Point
to declare variables of this struct type.
4. Advantages of typedef
Here are some advantages of using typedef
:
-
Code Clarity:
typedef
allows you to create more descriptive and self-explanatory type names, making your code easier to read and understand. -
Abstraction: It provides a level of abstraction by hiding the underlying implementation details of complex data structures. For example, you can use
typedef
to define custom data types for opaque data structures in libraries. -
Portability: Using
typedef
can improve code portability, as it makes it easier to change the underlying data type in one place without having to modify the rest of the codebase.
5. Example Programs
Let's explore a few examples to demonstrate the use of typedef
:
Example 1: Creating Custom Data Types
#include
typedef int Distance; // Create a custom type name "Distance" for integers
int main() {
Distance d1 = 10;
Distance d2 = 20;
printf("Distance 1: %d\n", d1);
printf("Distance 2: %d\n", d2);
return 0;
}
In this example, we use typedef
to create a custom data type name "Distance" for integers. This provides a more meaningful name for variables representing distances.
Example 2: Creating a Struct with typedef
#include
typedef struct {
int x;
int y;
} Point; // Create a new type name "Point" for an anonymous struct
int main() {
Point p1 = {3, 4};
Point p2 = {1, 2};
printf("Point 1: (%d, %d)\n", p1.x, p1.y);
printf("Point 2: (%d, %d)\n", p2.x, p2.y);
return 0;
}
In this example, we create a new type name "Point" for an anonymous struct. This makes the code more readable and allows us to create variables of type "Point" to represent 2D points.
typedef
is a valuable feature in C that enhances code readability and maintainability by allowing you to create meaningful names for data types, especially when working with complex structures or when you want to improve the self-documenting nature of your code.