Arrays in C: Building Blocks of Data Organization and Manipulation
Arrays in C are a cornerstone of programming, serving as a fundamental data structure that allows you to organize and manage collections of elements efficiently
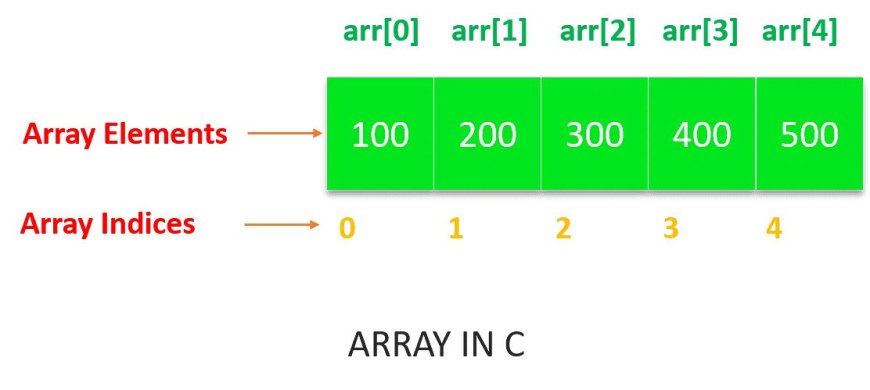
Arrays are a cornerstone of programming, serving as a fundamental data structure that allows you to organize and manage collections of elements efficiently. In the context of the C programming language, arrays play a vital role in various programming tasks, from simple data storage to complex algorithm implementation. In this article, we'll delve into the world of arrays in C, exploring their syntax, usage, and practical examples.
Understanding Arrays
At its core, an array is a contiguous block of memory that stores a fixed number of elements, all of the same data type. Each element is accessed by an index, which starts at 0 for the first element and increments sequentially. Arrays provide a systematic way to manage data, making it easier to perform operations on multiple values simultaneously.
Declaring and Initializing Arrays
In C, arrays are declared by specifying the data type followed by the array name and the number of elements enclosed in square brackets. Here's an example:
int numbers[5]; // Declares an array of 5 integers
You can also initialize arrays during declaration:
int primes[5] = {2, 3, 5, 7, 11}; // Initializes the array with values
Accessing Array Elements
Array elements are accessed using their indices:
int secondPrime = primes[1]; // Accesses the second element (3)
Looping Through Arrays
Loops are often used to iterate through arrays. For instance, a for
loop can be used to traverse the entire array:
for (int i = 0; i < 5; i++) {
printf("%d\n", primes[i]); // Prints each prime number
}
Array Bounds and Memory
C arrays don't have built-in bounds checking, so it's essential to ensure that you don't access elements beyond the array's bounds. Accessing elements outside the valid index range can lead to undefined behavior or memory corruption.
Example: Finding the Maximum Element
Let's use an array to find the maximum element within it:
#include
int main() {
int values[] = {42, 17, 99, 88, 64};
int max = values[0];
for (int i = 1; i < 5; i++) {
if (values[i] > max) {
max = values[i];
}
}
printf("Maximum value: %d\n", max);
return 0;
}
Multidimensional Arrays
C supports multidimensional arrays, which is a natural extension of the one-dimensional arrays in C. They allow you to organize data in a grid-like structure, making them particularly useful for tasks involving tabular or matrix-like data. In this article, we'll dive into the concept of multidimensional arrays in the C programming language, exploring their syntax, advantages, and practical examples.
Understanding Multidimensional Arrays
A multidimensional array in C can be thought of as an array of arrays. While a one-dimensional array is a linear collection of elements, a two-dimensional array introduces the concept of rows and columns, forming a grid or matrix.
Declaring Multidimensional Arrays
To declare a two-dimensional array, you specify the data type, followed by two sets of square brackets indicating the number of rows and columns:
int matrix[3][3]; // Declares a 3x3 integer matrix
You can initialize a multidimensional array during declaration, similar to a one-dimensional array:
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
Accessing Elements
To access elements in a two-dimensional array, you use row and column indices:
int value = matrix[1][2]; // Accesses the element in the second row and third column (6)
Nested Loops for Traversal
Nested loops are commonly used to traverse through multidimensional arrays. One loop iterates over rows, and the other loop iterates over columns:
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
printf("%d ", matrix[i][j]); // Prints each element
}
printf("\n"); // Moves to the next row
}
Applications of Multidimensional Arrays
Multidimensional arrays have a wide range of applications:
-
Matrices: Multidimensional arrays are ideal for representing mathematical matrices used in linear algebra operations.
-
Images: In image processing, images can be represented as arrays of pixel values, where each element corresponds to a pixel's color or intensity.
-
Tabular Data: Multidimensional arrays can model tabular data, such as a spreadsheet, where rows represent records and columns represent attributes.
-
Board Games: Game boards like chess or tic-tac-toe can be represented using multidimensional arrays.
Example: Matrix Multiplication
Let's implement matrix multiplication using multidimensional arrays:
#include
int main() {
int A[2][2] = {{1, 2}, {3, 4}};
int B[2][2] = {{5, 6}, {7, 8}};
int result[2][2] = {{0, 0}, {0, 0}};
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 2; j++) {
for (int k = 0; k < 2; k++) {
result[i][j] += A[i][k] * B[k][j];
}
}
}
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 2; j++) {
printf("%d ", result[i][j]);
}
printf("\n");
}
return 0;
}
Conclusion
Arrays in C enable the efficient storage and retrieval of data elements, making them a crucial tool for a wide range of programming tasks. Understanding how to declare, initialize, access, and manipulate arrays is essential for any programmer seeking to harness the full power of C. By mastering arrays, you lay the foundation for more complex data structures and algorithms, unlocking the potential to create robust and efficient software solutions.