File I/O Operations in C
File Input/Output (I/O) operations in C are essential for reading data from files and writing data to files. This tutorial will cover the basics of file I/O operations in C
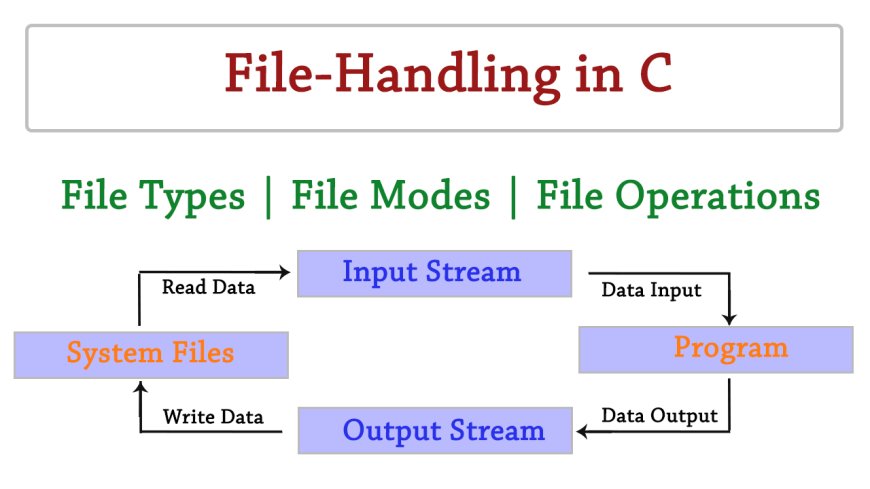
File Input/Output (I/O) operations in C are essential for reading data from files and writing data to files. This tutorial will cover the basics of file I/O operations in C, including opening, reading, writing, and closing files. We'll also provide examples to illustrate these concepts.
Opening a File
Before performing any file I/O operations, you need to open the file. The fopen
function is used for this purpose. It takes two arguments: the file name and the mode.
"r"
: Read mode"w"
: Write mode (creates a new file or truncates an existing one)"a"
: Append mode (creates a new file or appends to an existing one)"rb"
: Read mode in binary"wb"
: Write mode in binary"ab"
: Append mode in binary
#include
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "w"); // Opens or creates a file for writing
if (filePointer == NULL) {
perror("File opening failed");
return 1;
}
// File operations go here
fclose(filePointer); // Close the file when done
return 0;
}
Reading from a File
To read data from a file, you can use functions like fscanf
or fgets
for text files and fread
for binary files. Here's an example using fgets
:
#include
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "r");
if (filePointer == NULL) {
perror("File opening failed");
return 1;
}
char line[100];
while (fgets(line, sizeof(line), filePointer) != NULL) {
printf("%s", line);
}
fclose(filePointer);
return 0;
}
Writing to a File
To write data to a file, you can use functions like fprintf
or fputs
for text files and fwrite
for binary files. Here's an example using fprintf
:
#include
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "w");
if (filePointer == NULL) {
perror("File opening failed");
return 1;
}
fprintf(filePointer, "Hello, File I/O!\n");
fprintf(filePointer, "This is a new line.");
fclose(filePointer);
return 0;
}
Closing a File
It's crucial to close a file when you're done with it using the fclose
function. Failing to do so may result in data loss or resource leaks.
#include
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "r");
if (filePointer == NULL) {
perror("File opening failed");
return 1;
}
// File operations
fclose(filePointer); // Close the file when done
return 0;
}
Error Handling
File I/O operations can fail due to various reasons like the file not existing, lack of permissions, or disk full. It's essential to handle errors properly using conditional statements and functions like perror
or fprintf
to provide meaningful error messages.
Example Programs
Let's look at a couple of complete examples to demonstrate file I/O operations:
Example 1: Reading from a Text File
#include
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "r");
if (filePointer == NULL) {
perror("File opening failed");
return 1;
}
char line[100];
while (fgets(line, sizeof(line), filePointer) != NULL) {
printf("%s", line);
}
fclose(filePointer);
return 0;
}
In this example, we open a text file called "example.txt" in read mode, read its content line by line using fgets
, and print each line to the console.
Example 2: Writing to a Text File
#include
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "w");
if (filePointer == NULL) {
perror("File opening failed");
return 1;
}
fprintf(filePointer, "Hello, File I/O!\n");
fprintf(filePointer, "This is a new line.");
fclose(filePointer);
return 0;
}
In this example, we open or create a text file called "example.txt" in write mode, write two lines of text to it using fprintf
, and then close the file.
These examples cover the basics of file I/O operations in C. Remember to handle errors, close files when done, and choose the appropriate file mode (text or binary) based on your needs.