Bit Fields in C
Bit fields are used when you want to conserve memory by allocating specific, fixed numbers of bits for individual data members within a struct or union.
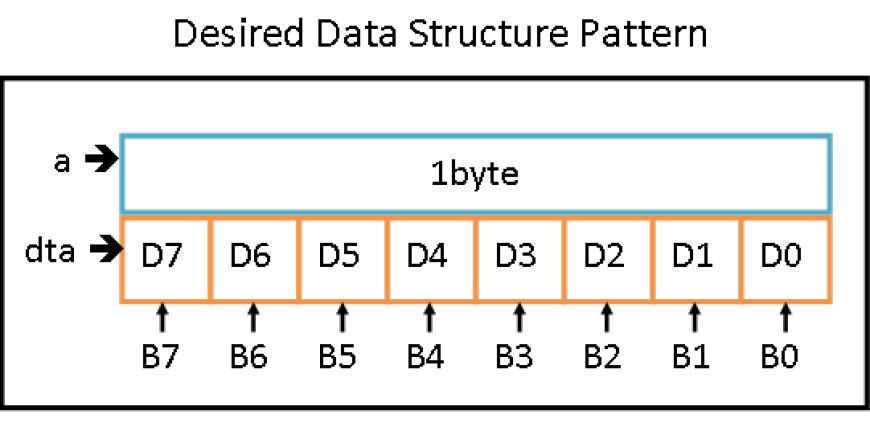
Bit fields in C are a feature that allow you to specify the number of bits a particular data member should occupy within a struct or union. This tutorial will explain the concept of bit fields, their syntax, and provide examples to illustrate their use.
1. Introduction to Bit Fields
Bit fields are used when you want to conserve memory by allocating specific, fixed numbers of bits for individual data members within a struct or union. They are especially useful in embedded systems and situations where memory efficiency is critical.
Bit fields allow you to define how much storage each data member should use, which is essential when dealing with hardware registers or communication protocols where data must be packed tightly.
2. Bit Fields Syntax
To declare a bit field in a structure, you use a colon :
followed by the number of bits you want to allocate to that data member. The syntax is as follows:
struct BitFieldStruct {
data_type member_name : num_bits;
};
data_type
: The data type of the bit field member (e.g.,int
,unsigned int
,char
, etc.).member_name
: The name of the bit field member.num_bits
: The number of bits you want to allocate to the member.
Here's an example:
struct Flags {
unsigned int isFlag1 : 1; // 1 bit for isFlag1
unsigned int isFlag2 : 1; // 1 bit for isFlag2
unsigned int value : 4; // 4 bits for value
};
In this example, we've defined a struct Flags
with three bit field members: isFlag1
, isFlag2
, and value
. isFlag1
and isFlag2
each occupy 1 bit, while value
occupies 4 bits.
3. Accessing Bit Fields
Bit fields are accessed just like regular members of a struct. You can read or write their values using the dot .
operator:
struct Flags myFlags;
myFlags.isFlag1 = 1; // Set isFlag1 to 1
myFlags.isFlag2 = 0; // Set isFlag2 to 0
myFlags.value = 7; // Set value to 7
printf("isFlag1: %u\n", myFlags.isFlag1);
printf("isFlag2: %u\n", myFlags.isFlag2);
printf("value: %u\n", myFlags.value);
4. Example Programs
Let's explore a few examples to demonstrate the use of bit fields:
Example 1: Packing Status Flags
#include <stdio.h>
struct StatusFlags {
unsigned int flag1 : 1;
unsigned int flag2 : 1;
unsigned int flag3 : 1;
};
int main() {
struct StatusFlags status;
status.flag1 = 1;
status.flag2 = 0;
status.flag3 = 1;
printf("Flag 1: %u\n", status.flag1);
printf("Flag 2: %u\n", status.flag2);
printf("Flag 3: %u\n", status.flag3);
return 0;
}
In this example, we define a StatusFlags
struct with three bit fields (flag1
, flag2
, and flag3
) to represent binary status flags. We then set and print their values.
Example 2: Packed RGB Color
#include <stdio.h>
struct RGBColor {
unsigned int red : 3;
unsigned int green : 3;
unsigned int blue : 2;
};
int main() {
struct RGBColor color;
color.red = 5;
color.green = 6;
color.blue = 3;
printf("Red: %u\n", color.red);
printf("Green: %u\n", color.green);
printf("Blue: %u\n", color.blue);
return 0;
}
In this example, we define an RGBColor
struct with three bit fields (red
, green
, and blue
) to represent the red, green, and blue components of a color. Each component is allocated a specific number of bits, and we set and print their values.
Bit fields in C are a powerful tool for optimizing memory usage in situations where you need to pack data tightly. They allow you to specify the number of bits each data member should occupy, providing fine-grained control over memory allocation. However, keep in mind that the number of bits allocated should not exceed the size of the data type, and the behavior is implementation-dependent for bit fields that span multiple bytes.