Operator Precedence and Associativity in C Language
Operators in programming languages are symbols that perform operations on one or more operands. When expressions involve multiple operators,
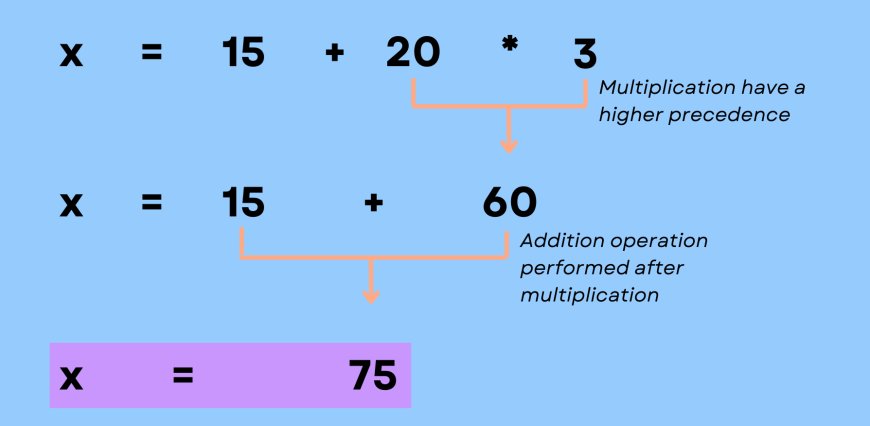
Operators in programming languages are symbols that perform operations on one or more operands. When expressions involve multiple operators, it's crucial to understand how these operators are evaluated. Operator precedence and associativity play a vital role in determining the order of evaluation in such cases.
Operator Precedence:
Operator precedence refers to the order in which operators are evaluated within an expression. Operators with higher precedence are evaluated before operators with lower precedence. C has a well-defined set of precedence levels for its operators. For instance:
- Postfix operators (
x++
,x--
) - Unary operators (
+x
,-x
,!x
,~x
,++x
,--x
,sizeof
,(type)
) - Multiplicative operators (
*
,/
,%
) - Additive operators (
+
,-
) - Shift operators (
<<
,>>
) - Relational operators (
<
,<=
,>
,>=
) - Equality operators (
==
,!=
) - Bitwise AND (
&
) - Bitwise XOR (
^
) - Bitwise OR (
|
) - Logical AND (
&&
) - Logical OR (
||
) - Conditional (Ternary) Operator (
?:
) - Assignment operators (
=
,+=
,-=
etc.) - Comma operator (
,
)
For example, in the expression a + b * c
, the multiplication operator *
has higher precedence than the addition operator +
, so b * c
is evaluated first, and then the result is added to a
.
Operator Associativity:
When multiple operators of the same precedence appear in an expression, associativity determines their order of evaluation. Associativity can be either left-to-right or right-to-left.
- Left-to-right associativity means that the leftmost operator is evaluated first.
- Right-to-left associativity means that the rightmost operator is evaluated first.
For example, in the expression a = b = c
, the assignment operator =
has right-to-left associativity. As a result, b = c
is evaluated first, and then the value of b
is assigned to a
.
Examples:
- Operator Precedence:
int result = 5 + 4 * 3; // Here, multiplication (*) has higher precedence than addition (+). // 4 * 3 is evaluated first, then added to 5, resulting in 17.
- Operator Associativity:
int a = 10, b = 5, c = 2; int result = a * b / c; // Here, multiplication (*) and division (/) have the same precedence. // Left-to-right associativity means a * b is evaluated first, then the result is divided by c. // The result is 25 / 2, which is 12.
Combining Precedence and Associativity:
int x = 5, y = 10, z = 15;
int result = z / y * x; // Division (/) and multiplication (*) have the same precedence.
// Left-to-right associativity means z / y is evaluated first, then the result is multiplied by x.
// The result is 1 * 5, which is 5.
In summary, understanding operator precedence and associativity is essential for writing accurate and predictable code. It ensures that expressions are evaluated in the desired order and helps avoid unexpected results.
Remember, if the order of evaluation is not clear in a complex expression, using parentheses can help explicitly specify the order. Always consult the documentation or a reliable resource to check the precedence and associativity of operators in the programming language you're working with.